Here is the interview question prompt, presented for reference.
Get ready to sail through a classic problem: Removing the n
th node from the end of a linked list in a single pass. By the end of this lesson, you'll be able to wave goodbye to that pesky n
th node and still maintain the list's structure.
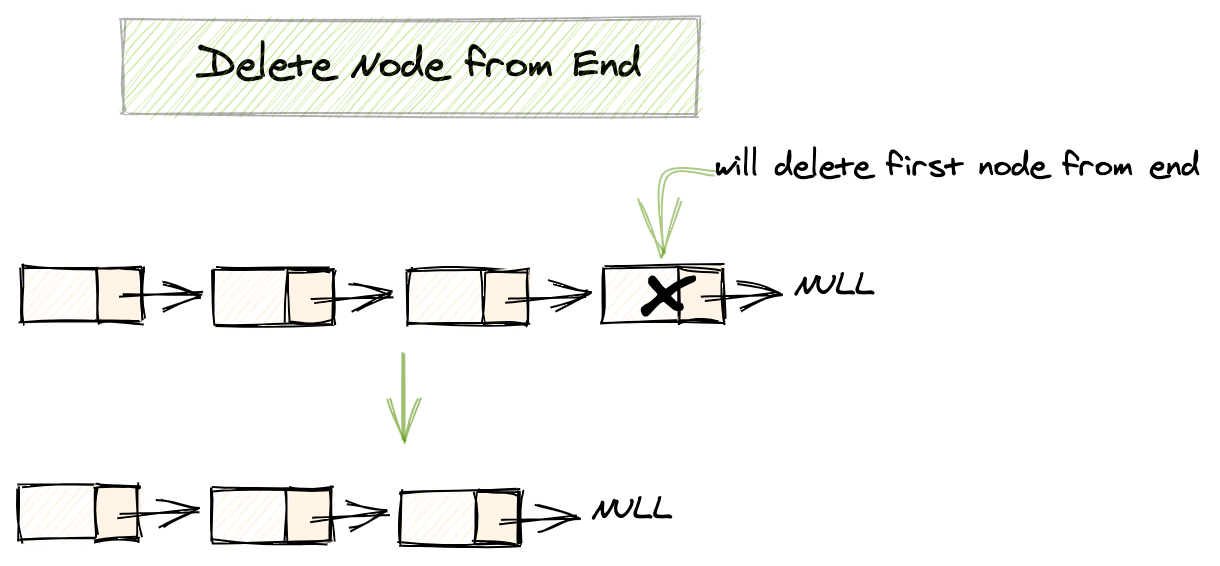
Imagine a chain of dominoes, each representing a node in our linked list. In this scenario, a list might look like this:
1 -> 2 -> 3 -> 4 -> 5
Your mission is to remove the n
th domino from the end. So, if we call removeFromEnd(head, 2)
, the chain would lose the 4
domino, and the head would still be 1
.
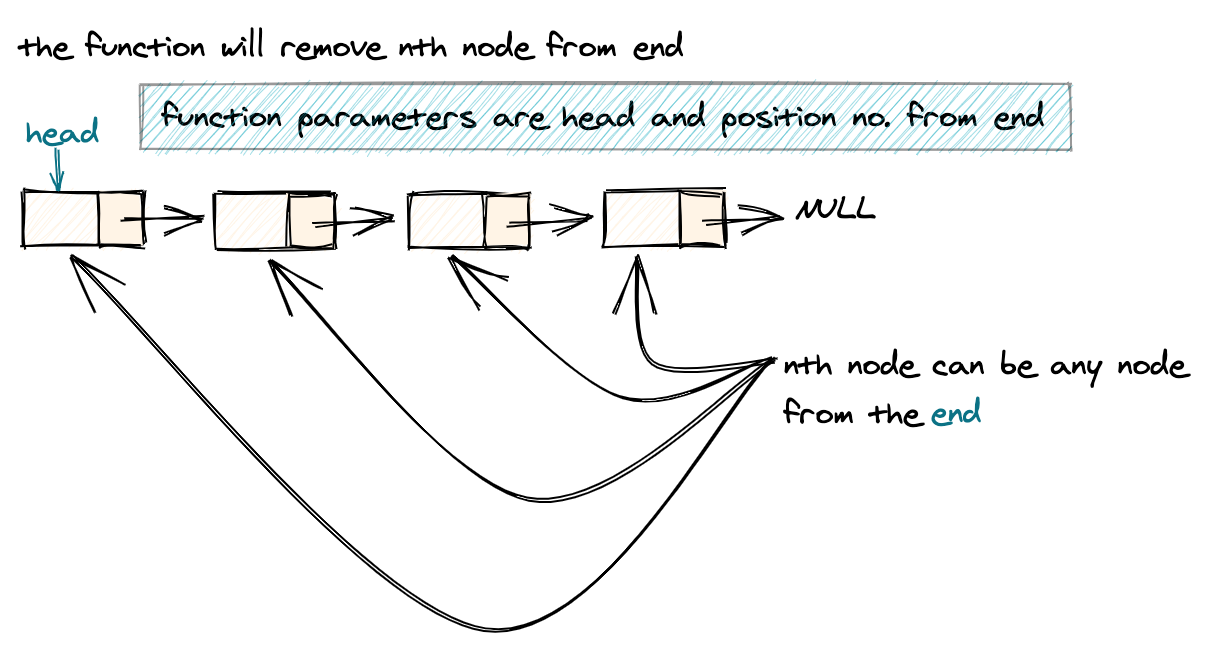
Before you jump in, let's set some ground rules:
n
exceeds the length of the list, then the chain remains intact. No dominoes are toppled!O(n)
and a space complexity of O(1)
.You can see the full challenge with visuals at this link.
Challenges • Asked over 7 years ago by Jake from AlgoDaily
This is the main discussion thread generated for Delete Node From End.