Here is the interview question prompt, presented for reference.
You are presented with two binary trees. Your task is to merge these trees into a single, new binary tree.
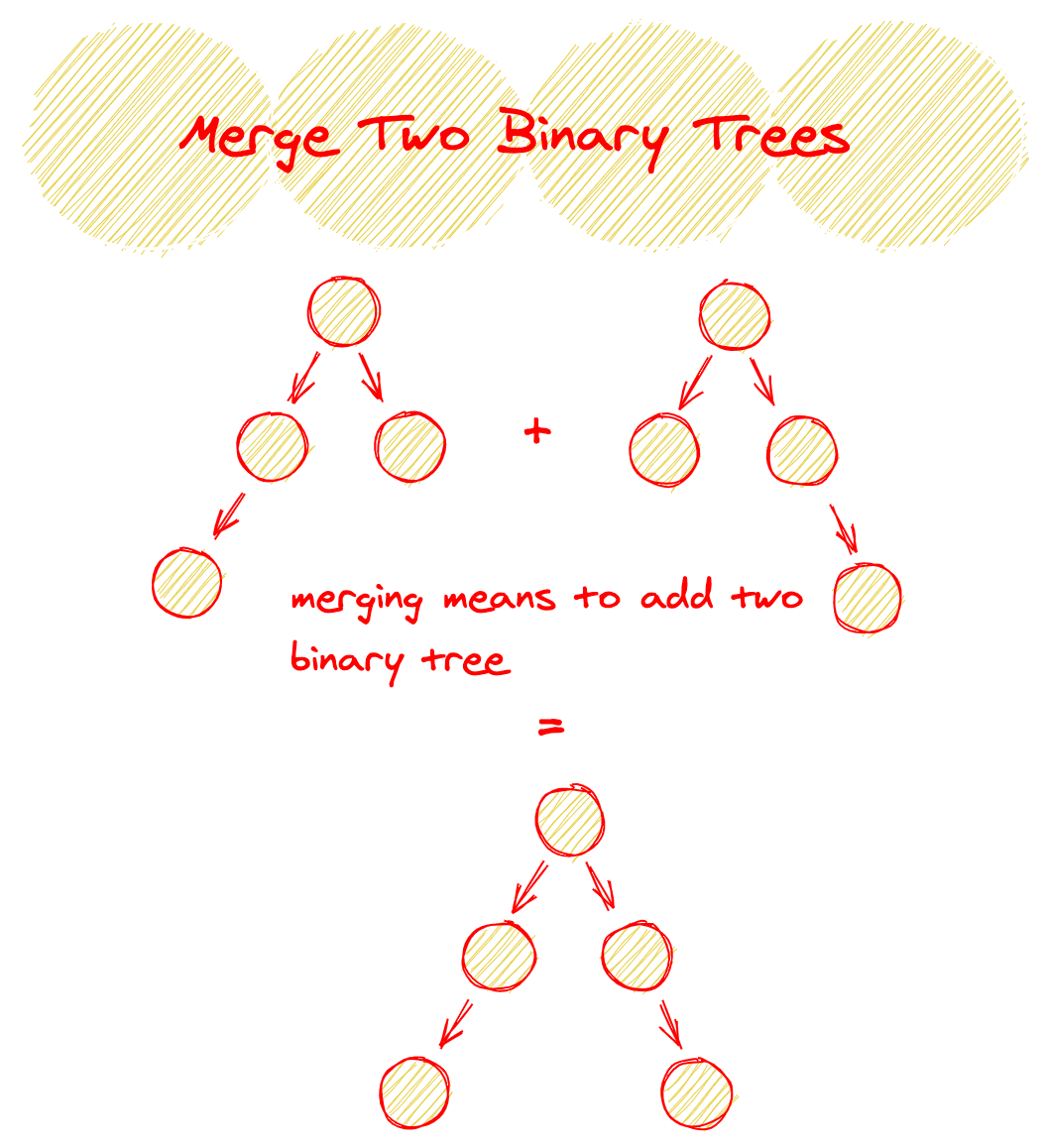
Here's the catch: some nodes in these two trees may overlap when you try to put them together. When that happens, the new node's value in the merged tree will be the sum of the overlapping nodes. For instance, consider the right leaf node in Tree 1 with a value of 2
and the right node in Tree 2 with a value of 3
. In the merged tree, the corresponding node would have a value of 5
(2 + 3).
If nodes don't overlap, the node value from the existing tree is simply carried over into the new tree.
Here's a quick example to make this more concrete:
Tree 1 Tree 2
1 2
/ \ / \
3 2 1 3
/ \ \
5 4 7
Resulting Merged Tree:
3
/ \
4 5
/ \ \
5 4 7
You can assume that the nodes in the tree are defined as follows in JavaScript:
function Node(val) {
this.val = val;
this.left = this.right = null;
}
100,000
nodes.-1,000,000,000
to 1,000,000,000
.Your task is to start merging from the root nodes of both trees and produce a new tree that satisfies the above conditions.
You can see the full challenge with visuals at this link.
Challenges • Asked over 7 years ago by Jake from AlgoDaily
This is the main discussion thread generated for Merge Two Binary Trees.