Here is the interview question prompt, presented for reference.
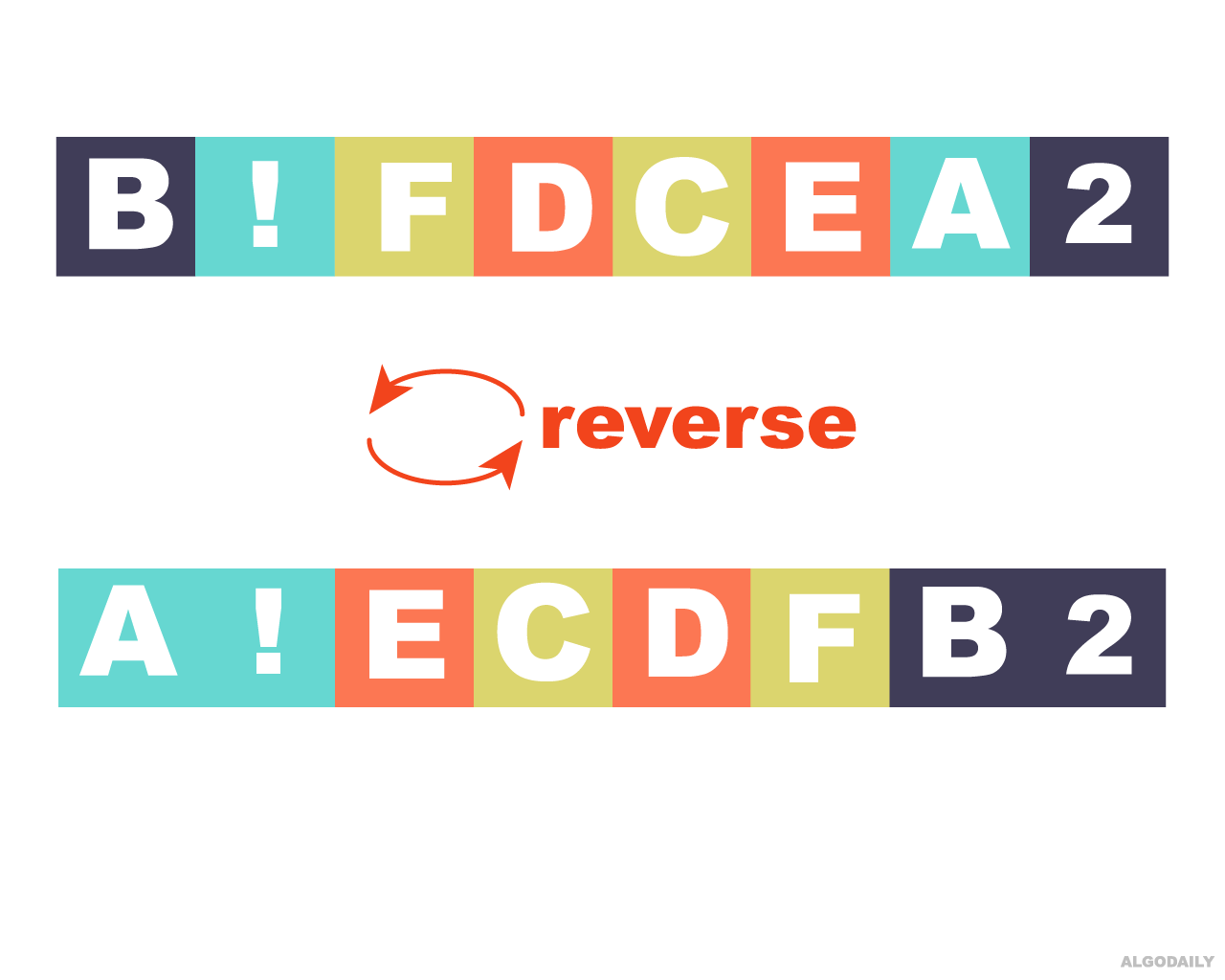
You are provided with a string that's a blend of alphabetic and non-alphabetic characters. Think of a beach where you find both shells (a-Z
) and other things like plastic wrappers or dollar bills ($, !, etc.
). For example, you might get:
'sea!$hells3'
Your mission, should you choose to accept it, is to flip only the alphabetical shells while keeping the other items ($, !, etc.
) in their original positions.
For example, when calling:
reverseOnlyAlphabetical('sea!$hells3');
You should expect the output to be:
'sll!$ehaes3'
100,000
characters.O(n)
.O(n)
.You can see the full challenge with visuals at this link.
Challenges • Asked almost 5 years ago by Jake from AlgoDaily
This is the main discussion thread generated for Reverse Only Alphabetical.
Hi @mattvanwinkle:disqus - thanks so much for catching this. I've updated the test cases to be correct.
For those reading-- in the original solution, in the second for-loop, I was not using a separator index to track the elements in reversedAlpha
. Hence, the letters were incorrectly placed. My bad!
I was a bit stumped by this one - shouldn't the the reversedAlpha array would have less indices than the original string if the original string contained characters?i.e. - input string - 'h!ey'reversedAlpha = ['y', 'e', 'h'];so on the 4th iteration over this string wouldn't it return undefined
for the character to swap?
update - ahh, I see! the solution you use has a separate index for that (as mentioned in your comment)
Hi, I'm using Python but it seems one can use the 2 pointer technique (as mentioned) for this problem. So the algorithm would be something like:
This way you don't have to use a regular expression, but I'm not sure of the time complexity? O(N)?
╷
├─ JUnit Jupiter ✔
└─ JUnit Vintage ✔
└─ MainTest ✔
├─ firstTest ✘ expected: but was:
└─ secondTest ✘ expected: but was:
Isn't the function supposed to return a single string? The characters are in the correct order but the tests fail due to difference in representation.. (I am returning a String as it is set by default return type).
I think using regex slows down this solution quite a lot.
I use the double-pointer method on the original string, only swapping if they are both alpha, and otherwise just incrementing/decrementing the index that is non-alpha.
For the 3rd test case this is about 13x faster than the main solution:
def reverse_alphabet_only(s):
output_str = list(s)
start = 0
end = len(s) - 1
for i in range(len(s)):
char1 = s[start]
char2 = s[end]
if char1.isalpha() and char2.isalpha():
output_str[start] = char2
output_str[end] = char1
end -= 1
start += 1
elif char1.isalpha() and not char2.isalpha():
end -= 1
elif char2.isalpha() and not char1.isalpha():
start += 1
else:
end -= 1
start +=1
if end <= start:
return "".join(output_str)