Here is the interview question prompt, presented for reference.
Imagine a family tree, but instead of tracking your relatives, it's tracking numbers. Each "person" in this tree has at most two "children," and some have none. We call these childless nodes "leaves," and your task is to find the sum of leaves that are on the right side of their "parent."
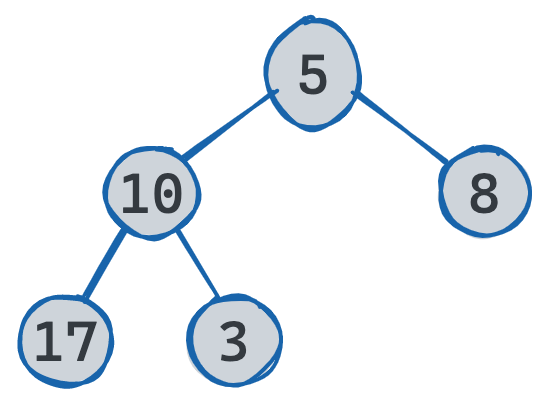
In this illustration, the right leaves are 8 and 3. Their sum would be 11.
Before diving into the solution, let's first establish what a Node in this tree looks like. We assume all nodes, even the root, follow this structure:
class Node {
constructor(val) {
this.value = val;
this.left = null;
this.right = null;
}
}
You need to implement the following function:
function sumOfRightLeaves(root) {
return root;
}
The function takes in the root node of the tree as an argument. Your goal is to traverse the tree and sum up all the right leaves.
So, how do we do that? Let's dive into the solution next.
You can see the full challenge with visuals at this link.
Challenges • Asked almost 7 years ago by Jake from AlgoDaily
This is the main discussion thread generated for Sum Right Side Leaves.