Here is the interview question prompt, presented for reference.
In this challenge, your task is to implement a queue using two stacks. While many programming languages offer queues through arrays or lists, we're restricting our approach to only utilize stacks.
The end result should behave like this:
const tsq = new TwoStackQueue();
tsq.push(1);
tsq.push(2);
tsq.pop(); // Should return 1
tsq.pop(); // Should return 2
Imagine having two stacks that work in harmony to simulate a queue. These stacks are like two components of a complex machine, each with its own specific role.
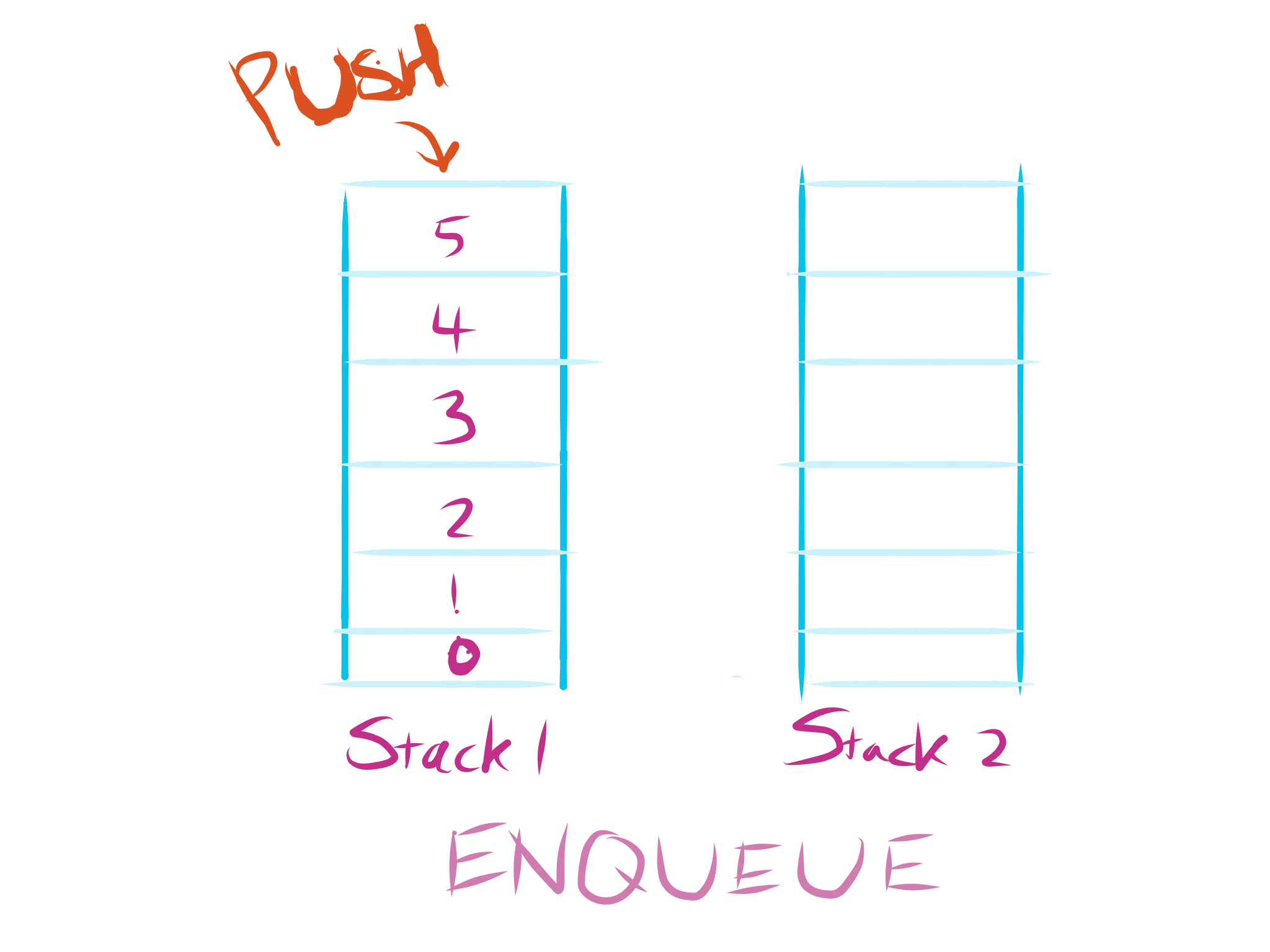
Let's clarify the rules of the game:
O(n)
, then the push operation should be O(1)
. Otherwise, the time complexity for push should be O(n)
.O(n)
, then the pop operation should be O(1)
. Otherwise, it should be O(n)
.O(n)
.You can see the full challenge with visuals at this link.
Challenges • Asked over 7 years ago by Jake from AlgoDaily
This is the main discussion thread generated for Two Stack Queue.
Test case 3 is wrong
Hi Aishwarya,
For which language?
For python
According to test 3
tsq = TwoStackQueue(); tsq.push(1); tsq.push(2); tsq.pop(); tsq.push(3); tsq.pop(); assert tsq.pop() == 1
tsq should pop 1 but it pop 3 since the operation order is push,push,pop,push,pop,pop